Getting Started: How to Set up a Full-Stack App with AWS and Bedrock
The AWS Bedrock Tutorial I Wish I Had: Everything You Need to Know to Prepare Your Machine for AWS Infrastructure
Part 1: Save countless hours of dealing with fragmented and incomplete documentation and have your environment ready in less than one hour
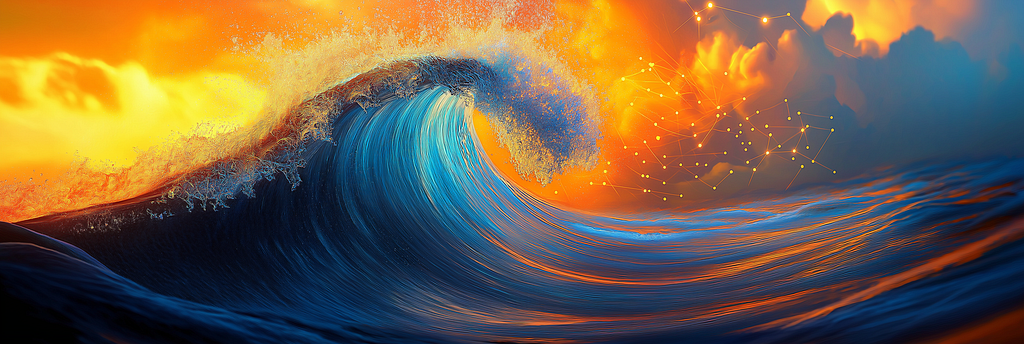
How can you take a nifty little machine-learning prototype in your notebook and develop it into a powerful full-stack web application? While this process might seem daunting, this multi-part series will help you tackle the learning curve one step at a time, guiding you through even the most tricky permissions issues (for which AWS is infamous). By the end of this series, you will have a fully functional language translation app to tinker with and have built the conceptual knowledge required to scale your own GenAI pipeline quickly.
This is part 1 of our new multi-part series on 🌊 An Extensive Starting Guide To Building Full-Stack GenAI Apps Powered by Amazon Bedrock.
This article series has been written in collaboration with Vlad Seredniy.
What is this series about?
Developing a full-stack application with Amazon Bedrock can present a fairly intense learning curve. A new developer is dealing with the complexities of AWS infrastructure and all of the intricacies of integrating an AI pipeline. Trying to learn the AWS tech stack can easily become quite frustrating, especially when dealing with fragmented documentation and the time-consuming process of customizing data flow between front-end and back-end systems. It’s not uncommon for developers to spend hours watching conceptual videos about the AWS stack with only limited practical applications.
In this series, we’re tackling these challenges head-on. We will teach you how to build a full-stack AI app using AWS infrastructure with React, Node JS, and Amazon Bedrock — step by step, with full understanding and (hopefully) minimal overwhelm.
We will be using this tutorial as a starting point. We will fill in all the gaps to help get you running, starting from how to set up the stack (in this tutorial) and then moving on to understanding the app codebase and adding new features like audio transcription, translation, and generation — essentially implementing custom AI pipelines. From simplifying the AWS setup and demystifying permissions to offering a comprehensive code overview of how the front end, back end, and Lambda functions work together, we aim to reduce friction and lower the barriers to full-stack integration. Along the way, we will try to thoroughly answer all the “silly” questions one could be afraid to ask in a work setting. At the end of this process, we hope to provide you with all the tools and confidence to pursue your own AI ideas and creations!
This series is based off of this project: AWS AppSync AI Agent Playground and this tutorial: Create a Fullstack, Sample Web App powered by Amazon Bedrock
In Part 1 (this part) of this series, we’ll walk through the essential steps to set up your AWS infrastructure and get a sample full-stack app running using Amazon Bedrock. We will cover how to create your AWS account, offer a video on configuring IAM users and getting secret keys, and install tools like Node.js and AWS CDK. By completing this first part, you’ll have a fully configured environment ready for customization.
In Part 2, we’ll address one of the biggest challenges new developers face when getting started with the AWS stack: the lack of clear documentation. We will show you around the project's frontend codebase and detail how we created components in React to capture and upload an audio file to an s3 bucket for processing.
When we talk with developers, they will cite permissions as of the most challenging aspects of using AWS infrastructure. In this part of the series, we will cover tricky AWS configuration details, such as setting up IAM roles and policies and configuring CORS for your s3 bucket.
Part 3 is where the fun really begins! We will hack into one of the agents in the sample project’s back-end Python files to integrate our GenAI tools. Specifically, we will utilize AWS Transcribe to transcribe audio and Bedrock’s capabilities to query Anthropic’s Claude API to translate the text from the transcription process.
Finally, in the series' conclusion, in Part 4, we will interface with Deepgram’s AI-powered voice generation API to produce a translated audio file that can be played in the app’s interface.
When you complete this series, you can expect a working full-stack AI-powered language translation application and an understanding of how to customize it with your own GenAI tools.
When is AWS Infrastructure the Right Choice for Your Project?
Obviously, the answer to this question goes beyond the scope of this article. However, we want to touch on the topic briefly by distinguishing between prototyping, testing an app, and deploying it at scale.
From our experience, when we are starting, during the learning or tinkering phase, it’s often a lot quicker to use tools like Jupyter Notebook, Google Colab, or even Lightning AI to test and iterate ideas in real time. Once we have chosen the technology stack we want to use and have the core functionality in place (and technical issues resolved), we build a bare-bones prototype. At this stage, we might use a more straightforward system (than AWS) such as Flutterflow or Streamlit.
However, when an app needs to handle increased traffic or robust security features, AWS has an enormous advantage as it can scale seamlessly as infrastructure needs grow.
Streamlined AWS Configuration
The remainder of this article will focus on setting up the sample AWS Bedrock app properly and getting it to run locally on your development machine. This part can be particularly tricky due to the fragmentation of documentation on Amazon’s website. We will expand upon the instructions from the Prerequisites section of the original tutorial and flesh out the steps to get all the requirements installed correctly.
📌 Note: sudo and Command Line Usage
Many of the upcoming sections require extensive use of your command line / terminal. If you happen to encounter permission errors, try prefixing your command with sudo.
For example, instead of usingnpm install -g aws-cdk, give sudo npm install -g aws-cdk a try.
Create your AWS IAM User
One of the first (and honestly most frustrating) parts for a beginner can be getting your AWS account set up with the right credentials. Below is a step-by-step process so you don’t have to spend hours bouncing around AWS’s website and documentation in an effort to piece together the correct sequence of actions.
Sign up for an AWS account.
Then log in and navigate to IAM in your console. Click on Users -> Create User
Now, you’ll need to customize the following details as you step through the Create User flow.
Step 1: Specify User Details
- Check the box for Provide user access to the AWS Management Console
- Select I want to create an IAM user
- In the Console password section, select Custom password. Enter a secure and memorable password.
- Uncheck Users must require a new password at next login so the user doesn’t have to reset the password upon first login.
Step 2: Set Permissions
- Select Attach policies directly.
- In the search bar, type AdministratorAccess
- Check the box next to the AdministratorAccess policy in the search results.
Next, click Create user in Step 3 and click Return to users list in Step 4. Remember to save your password in a secure location.
After setting up your IAM user, you’ll need to install the required software tools, starting with Node.js.
Install Node and NPM (Node Package Manager)
You must already have NPM (Node Package Manager) installed to set up any of Amazon’s developer tools on your local machine. Our next step will be to install Node.js, which includes NPM. The sample project requires Node.js v18 or higher, so we will install the latest version to cover this dependency.
For Mac:
- Visit the official Node.js website. You can either copy and paste commands into your terminal to download and install via the command line, or simply download the installer for Mac.
For Linux (Ubuntu):
sudo apt install nodejs npm
Verify the installation:
node — version
npm — version
With Node.js installed, the next step is setting up the AWS Cloud Development Kit (CDK) with NPM.
Configure your AWS account on your dev machine
Now, we will show you how to use NPM to install AWS CDK. The sample app requires AWS CDK 2.103 or later so we will download the most recent version (which ensures this requirement is met).
Enter the following command to install AWS CDK globally:
sudo npm install -g aws-cdk
Let’s verify the installation:
cdk — version
With CDK installed, we can install AWS CLI (Command Line Interface), which will allow you to configure your AWS account credentials.
Installing and Configuring AWS CLI
Setting up your account credentials is essential to correctly setting up the sample app, so let’s cover how to install AWS CLI and set up your account correctly.
Installing AWS CLI
The method to install AWS CLI differs slightly depending on your operating system.
For Linux, download and run an installer:
curl “https://awscli.amazonaws.com/awscli-exe-linux-x86_64.zip" -o “awscliv2.zip”
unzip awscliv2.zip
sudo ./aws/install
For Mac, download the *.pkg file from the AWS CLI website and follow the instructions in the installation wizard.
Let’s wrap up this step by verifying that the installation worked:
aws — version
Creating Access Keys
Now that we have AWS CLI installed, we can move on to creating access keys. If you followed the instructions in the video from Step 1, you already have your access keys created. If not, go ahead and review the end of the video again or follow these directions:
- In the IAM dashboard, look for the user you want to create access keys for.
- In the user Summary section, click on “Create access key”
- Check “I understand the above recommendation and want to proceed to create an access key”
- Copy and paste your access key and secret access key in a safe location.
Configuring AWS CLI
We are almost there! With one last step, your account will be configured correctly on your development machine.
Run this command to launch the configuration process:
aws configure
You’ll be asked to enter the following:
- AWS Access Key ID (copy / paste from your secure location)
- AWS Secret Access Key (copy / paste from your secure location)
- Default region name (we used us-east-1)
- Default output format (use json)
Once this step is complete, your account details will be configured, and you can move on to getting ready to deploy AWS CDK infrastructure.
Bootstraping the CDK
Next, we will show you how to bootstrap your CDK in the region you wish to deploy.
cdk bootstrap aws://ACCOUNT-NUMBER/REGION
Here, you will need to use your 12 digit account number (you can find this at the top right of your screen on the AWS console) for ACCOUNT-NUMBER and your region (we use us-east-1) for REGION.
You’re almost there! Now you just need to install the additional tools for the project and you will have the sample app setup.
Installing Yarn, Git, and Docker
This project uses yarn to manage dependencies. You can install it with NPM:
sudo npm install -g yarn
You can verify that yarn installed correctly:
yarn — version
Git:
Git comes pre-installed on Mac and most Linux distributions. You can verify that it’s installed.
git — version
If it’s not already installed, on Ubuntu you can use apt-get sudo apt-get install git and on Mac it can be downloaded from the official Git website.
Docker:
On Mac, you can download Docker Desktop for Mac from the official Docker website and then just follow the installation wizard.
For Linux (Ubuntu), you can follow the official Docker installation guide for Ubuntu.
Conclusion
Congratulations! You’ve completed Part 1 of this series and should now have your development environment with all the dependencies set up so that you can deploy this AWS sample app. You can now pick up from the original tutorial's Setup section and download the project code using Git.
Next Steps:
AWS services and setup processes can always change, so even though we’ve tried to make these instructions as current as possible, you can always check the AWS documentation for the most up-to-date information if something isn't working.
We will follow up in the coming weeks to discuss in depth the juicy implementation details for adding all the custom functionality to make an interactive translation app. This will give you a chance to engage with the concepts and build an understanding of the tech stack.
👉 What you will find in part 2:
In Part 2, we will be zooming out and give you an overview of the sample app’s architecture. We will show you how we customized the front-end to capture and store a voice recording, and how to pass it through the API to the backend.
Getting Started: How to Set up a Full-Stack App with AWS and Bedrock was originally published in Towards Data Science on Medium, where people are continuing the conversation by highlighting and responding to this story.
from AI in Towards Data Science on Medium https://ift.tt/2HZq7BS
via IFTTT